Explore my C++ Projects
As a passionate programmer, I specialize in designing efficient and engaging games using C++ and SFML. With a strong foundation in data structures and algorithms, I have developed innovative projects that combine technical precision with creative gameplay. My C++ projects demonstrate mastery of concepts such as linked lists, arrays, and recursion, brought to life through dynamic visuals and smooth gameplay mechanics using SFML. Each project reflects my ability to solve complex problems and implement game logic that is both efficient and scalable. Explore my work to see how I turn core programming concepts into exciting interactive experiences.
Linked List Snake 2D

Linked List Snake 2D 🐍:
-
Genre: 2D Arcade/Strategy
-
Engine: C++ with SFML
-
✨ Highlights of the Game
-
🎮 Dynamic Gameplay: Designed unique food mechanics:
-
🍕 Junk Food: Pizza, burger, and cheese trigger Linked List insertions (head, middle, tail) to grow the snake.
-
🍎 Healthy Food: Apple, mango, and orange cause Linked List deletions (head, middle, tail) to shrink the snake.
-
💀 Poison: Cuts the snake in half instantly.
-
🍻 Alcohol: Reverses the snake's direction with Linked List reversal techniques.
-
-
⚡ Customization: Players can switch between Single and Doubly Linked List modes with real-time visualization of operations and time complexities.
-
🕹️ Challenging Levels: Added multiple levels with creative obstacles and layouts for engaging replayability.
-
📊 Real-Time Mechanics: Synced Linked List operations seamlessly with gameplay, enhancing the player experience.
-
🎥 Smooth Visuals: Integrated animations and immersive sound effects for polished gameplay.
-
-
🌟 Key Takeaways:
-
📈 Data Structures Mastery: Applied Linked Lists, STL vectors, and OOP principles for modular, scalable code.
-
🧩 Problem-Solving: Overcame challenges like syncing data structure operations with real-time mechanics.
-
🎨 Game Development Expertise: Strengthened skills in SFML for creating smooth visuals, animations, and level design.
-
👥 User-Centric Design: Focused on intuitive gameplay and customization options for diverse player preferences.
-
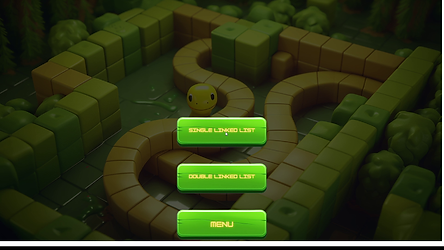

Sorting Sticks

Sorting Sticks 🎮✨:
-
Genre: Educational/Interactive Simulation
-
Engine: C++ with SFML
-
✨ Highlights of the Game
-
🧩 Algorithm Showcases: Brought sorting algorithms to life with visual demonstrations of Bubble Sort, Insertion Sort, Selection Sort, Merge Sort, Quick Sort, and Radix Sort.
-
🎨 Interactive Gameplay: Designed smooth animations, vibrant visuals, and engaging sound effects using SFML.
-
🛠️ Scalable Design: Applied clean OOP principles to ensure a modular and flexible architecture for future enhancements.
-
-
🌟 Key Takeaways:
-
💡 Sorting Algorithm Expertise: Gained hands-on understanding of time and space complexities, exploring the efficiency and best use cases of each algorithm.
-
⚙️ Dynamic Problem-Solving: Seamlessly integrated sorting algorithms into an interactive game environment, making technical concepts engaging and intuitive.
-
🎨Game Development Skills: Enhanced proficiency in C++ and SFML, focusing on creating polished animations and a smooth game flow.
-
📚 Educational Impact: Designed an intuitive platform to make learning sorting algorithms interactive, fun, and visually appealing.
-
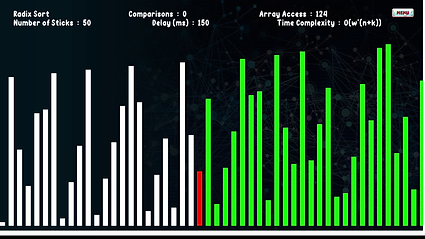
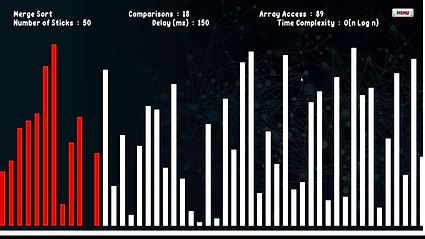
Searching Sticks

Searching Sticks 🎮✨:
-
Genre: Educational/Interactive Simulation
-
Engine: C++ with SFML
-
✨ Highlights of the Game
-
🔍 Search & Learn:
-
Linear Search Mode: Explore an unsorted list one stick at a time.
-
Binary Search Mode: Leverage the Binary Search Algorithm on a sorted list for faster and optimized searching.
-
-
🎨 Interactive Visuals: Each algorithm step is vividly animated, making learning intuitive and enjoyable.
-
🛠️ Clean Design: Built using Object-Oriented Programming principles to ensure scalability and flexibility for future improvements.
-
-
🌟 Key Takeaways:
-
💡 Algorithm Mastery:
-
Gained practical insights into Linear Search, exploring its efficiency for smaller or unordered datasets.
-
Mastered Binary Search, understanding its logarithmic time complexity and its importance for sorted data.
-
-
⚙️ Integration Expertise: Successfully implemented searching algorithms into an engaging game, blending education with entertainment.
-
🎨 Game Development Skills:
-
Advanced proficiency in C++ and SFML to deliver smooth animations and immersive sound effects.
-
-
📚 Educational Impact: Designed an engaging way to visualize searching algorithms, making complex concepts approachable and interactive.
-


2D Array Mine Sweeper

2D Array Mine Sweeper 💣:
-
Genre: 2D Puzzle/Strategy
-
Engine: C++ with SFML
-
Game Features 🎮
-
🎮 Classic Gameplay: Designed strategic and engaging Minesweeper mechanics that balance fun with frustration, offering a nostalgic experience.
-
🎨 Visuals & UI: Developed smooth rendering, interactive visuals, and satisfying sound effects, enhancing immersion with SFML.
-
🛠️ Clean Design: Implemented the MVC pattern for modular, scalable, and maintainable code structure.
-
🌌 Optimized Performance: Leveraged efficient 2D array operations and STL vectors to streamline gameplay logic.
-
-
Key Learnings🧠
-
📈 Data Structures Proficiency: Strengthened expertise in 2D arrays and STL vectors for advanced game logic.
-
🧩 OOP Expertise: Applied C++ Object-Oriented Programming principles to create a flexible and future-ready game design.
-
🎨 Game Development Skills: Mastered SFML for crafting polished visuals, smooth animations, and immersive sound effects.
-
👥 User-Centric Design: Focused on creating an intuitive and satisfying player experience.
-
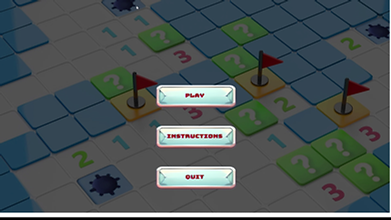
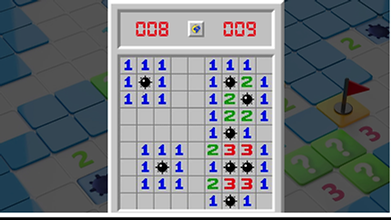
1D Array Jumper

1D Array Jumper 🎮:
-
Genre: 2D Puzzle/Platformer
-
Engine: C++ with SFML
-
✨ Highlights of the game:
-
🕹️ Strategic Player Movement: Players navigate the platform by moving or jumping based on numbers assigned to the 1D array tiles.
-
🧩 Puzzle Decisions: Designed gameplay requiring strategic choices—move forward, backward, or jump to avoid obstacles.
-
❤️ Health System: Players start with 3 lives; losing all sends them back to the start, increasing tension and replayability.
-
🚀 Level Progression: Introduced progressively tougher challenges and obstacles with each cleared level for added complexity.
-
🎨 Visuals & Sound: Leveraged SFML for smooth rendering, interactive visuals, and immersive sound effects.
-
-
🌟 Takeaways and Growth:
-
📈 Data Structures Expertise: Enhanced understanding of 1D arrays and STL vectors for gameplay logic.
-
🛠️ Design Patterns: Followed the MVC pattern to create clean, modular, and scalable code.
-
🎨 Game Dev Skills: Refined skills in SFML to deliver polished visuals and smooth gameplay mechanics.
-
👨💻 C++ OOP: Applied object-oriented programming principles for flexibility and future-proofing the codebase.
-
👥 User Engagement: Focused on creating a challenging yet enjoyable player experience.
-
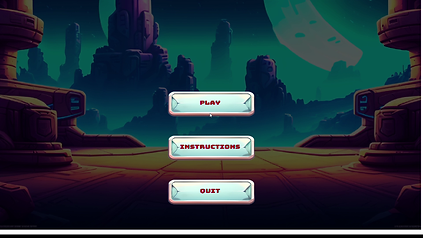

Pong

Pong 🎮✨:
-
Genre: Classic Arcade Game
-
Engine: C++ with SFML
-
✨ Highlights of the game:
-
🏓 Rendering Graphics: Designed game elements like paddles, ball, and background using SFML’s rendering capabilities.
-
⚡ Collision Detection: Implemented real-time detection for ball-paddle interactions, enhancing gameplay realism.
🔢 Score Tracking: Created a dynamic, real-time score display to maintain player engagement.
🎮 Smooth Controls: Enabled responsive paddle movement with real-time input handling for an immersive experience.
-
-
🌟 Takeaways and Growth:
-
💡 SFML Fundamentals: Mastered SFML for rendering graphics, handling input, and managing window events efficiently.
⚙️ Game Loop Design: Built a simple, yet robust game loop to drive mechanics and ensure fluid gameplay.
🎨 C++ OOP: Applied Object-Oriented Programming principles to create clean, modular, and scalable code.
-

